What is JavaScript Strict Mode and Why You Should Use It?
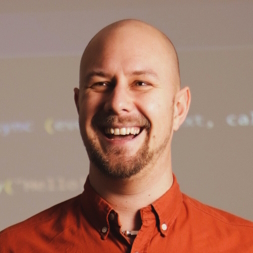
By James Charlesworth
3 December 2023
Explore the benefits of JavaScripts strict mode in enhancing code reliability and preventing common mistakes. This article delves into its features, real-world examples, and reasons why both beginners and pros should consider its adoption.
JavaScript's "strict mode" is a feature that helps developers write safer and more reliable code. When enabled, it changes some JavaScript behaviors that are considered error-prone and can prevent common coding mistakes. Think of it as a way to make your JavaScript code more robust without the need for a full compilation step, like in TypeScript.
In this article, we'll dive deep into strict mode, explore its benefits, and look at some real-world examples.
Enabling Strict Mode
Activating strict mode is straightforward. All you need to do is include the string "use strict"; at the beginning of your JavaScript file or function.
"use strict";
This line instructs the JavaScript engine to interpret and execute the code in strict mode. Keep in mind that this directive is only recognized at the beginning of a script or a function, so you must decide to use strict mode for the entire script.
How Strict Mode Improves Your Code
- Undeclared Variables Without strict mode, if you use a variable that hasn't been declared, JavaScript will automatically create it as a global variable, which can cause unexpected behavior and bugs.
However, when strict mode is enabled:
x = 10;
This line will throw a ReferenceError stating that x is not defined.
-
Disallowing the with Statement Strict mode prohibits the use of the with statement, considered bad practice. By doing so, it promotes clearer and more explicit code.
-
Extra Function Arguments In regular JavaScript, if you call a function with more arguments than it is defined to accept, the extra arguments are simply disregarded.
For instance:
function exampleFunc(arg1, arg2){
// some code
}
exampleFunc(1, 2, 3);
The third argument in the function call above would be ignored in standard mode. However, with strict mode enabled, it throws a SyntaxError, pointing out potential coding errors.
-
Writing to Read-Only Properties In non-strict mode, you can write to properties declared as writable: false. With strict mode, attempting such an action throws a TypeError.
-
Duplicate Property Names In standard JavaScript, if an object has duplicate property names, the last one prevails. With strict mode turned on, it will raise an error if you try to create an object with repeated property names.
Conclusion
JavaScript's strict mode is a way to embrace a more constrained version of the language, eliminating many silent errors that can arise in standard JavaScript. By enabling strict mode, you can identify potential bugs earlier, ensuring your code remains consistent and easier to maintain. Whether you're a beginner or a seasoned developer, strict mode offers a pathway to write better, less error-prone code.
So, if you're new to JavaScript, kick off your journey with strict mode to avoid bad coding habits. And if you're a pro, consider revisiting your existing projects and switching to strict mode for enhanced robustness.
Watch on YouTube
Check out this video on our YouTube channel about strict mode.
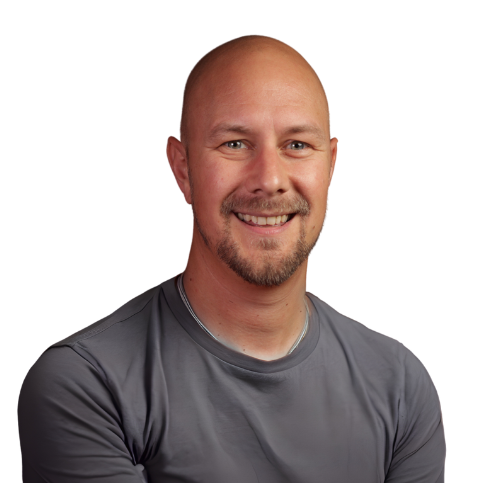
Hi, I'm James
I've worked in software development for nearly 20 years, from junior engineer to Director of Engineering. I'm here to help you get your foot on the next rung of your career ladder. I post weekly videos on YouTube and publish guides, articles, and guided project tutorials on this site. Sign up to my newsletter too for monthly career tips and insights in the world of software development.
Related Project Tutorials
Read Next...
How to Run TypeScript in VS Code
30 November 2023 • 3 min read
Learn how to set up, run, and debug TypeScript in Visual Studio Code. This guide provides step-by-step instructions to enhance your JavaScript development process. Dive into the seamless integration of TypeScript with VSCode for a productive coding session.
First Class Functions in Javascript
17 August 2023 • 3 min read
This article introduces you to first class functions, and how functions can be treated as first class citizens in programming, demonstrating their utility and practical applications in Javascript.
Simple marketing website with tailwind CSS and React
17 December 2022 • 14 min read
Complete guide to building a landing page for a SaaS application from scratch, using Vite + React + Tailwind